How to Add Custom Amount with PayPal Express Checkout in Magento 2
Vinh Jacker | 09-09-2024
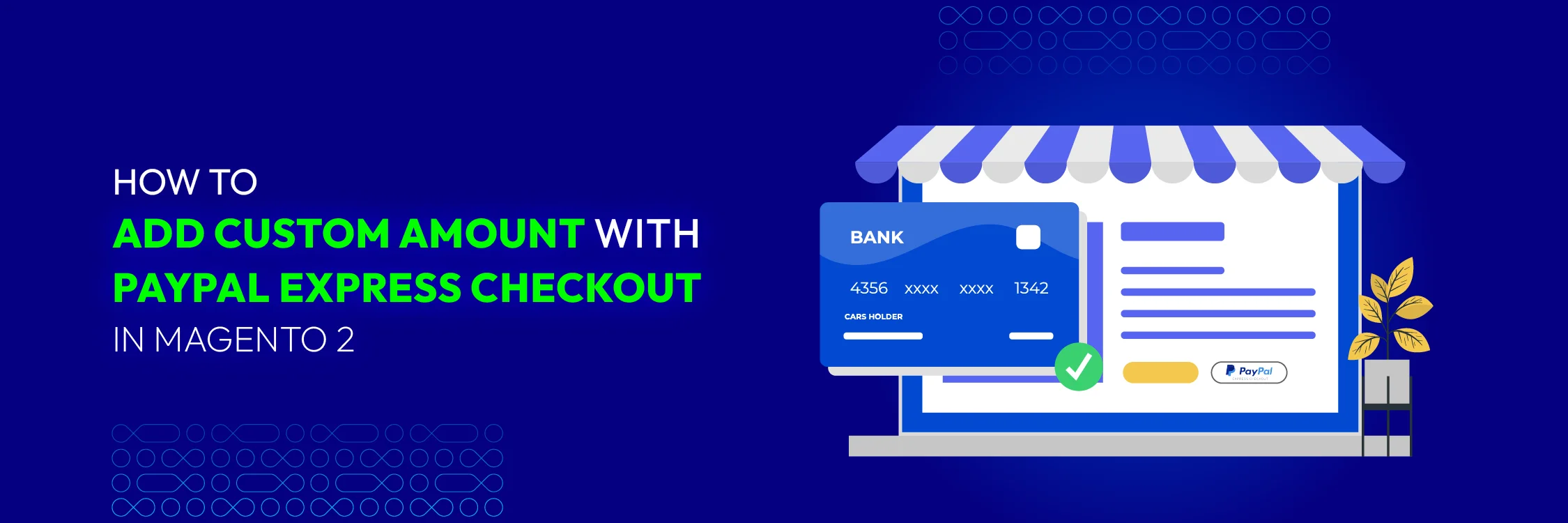
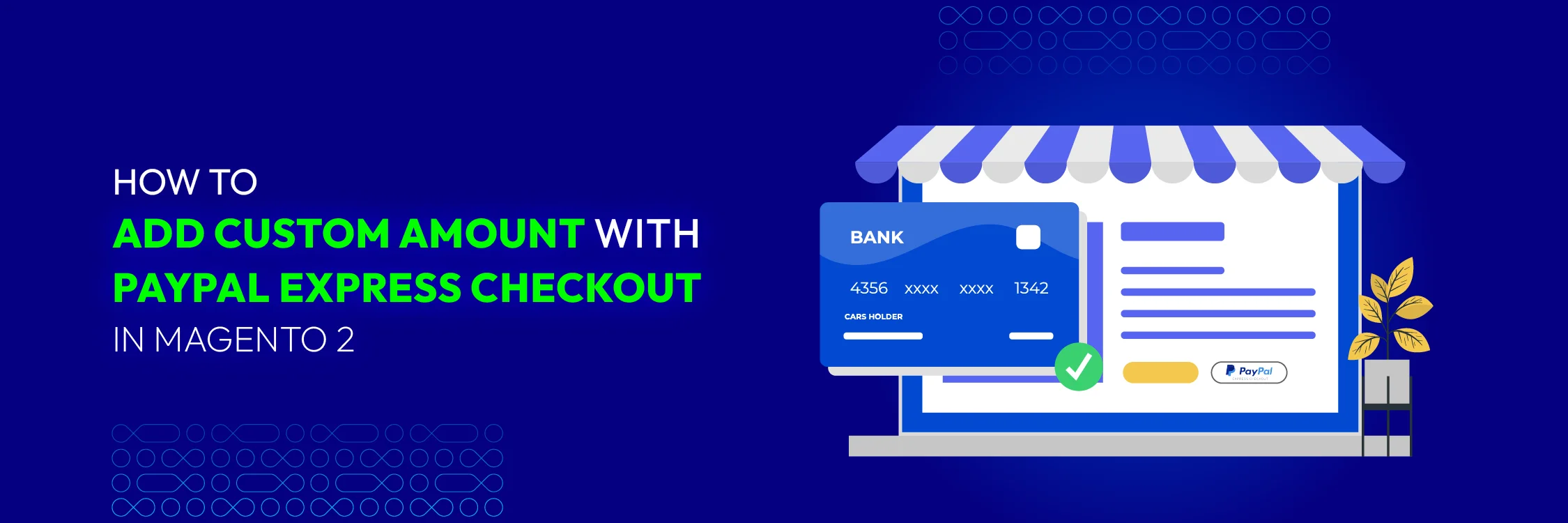
The Most Popular Extension Builder for Magento 2
With a big catalog of 224+ extensions for your online store
Adding a custom amount with PayPal Express Checkout in Magento 2 is essential when you want to charge extra fees or offer discounts based on specific conditions. This might include applying a handling fee, gift wrapping charge, or promotional discount.
In this article, we’ll explore two effective methods to add custom amount with PayPal express checkout in Magento 2: overriding the payment model and using an observer to customize the amount.
Things to Prepare Before Adding a Custom Amount with PayPal Express Checkout
First of all, you need a registered module. Then, to add the amount with PayPal Express Checkout in Magento 2, follow these steps with 2 methods.
Method 1: Override the Payment Model
Step1: Dependency Injection Configuration
Create file app/code/Vendor/Module/etc/di.xml
Add the following content to di.xml:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<preference for="Magento\Paypal\Model\Express\Checkout" type="Vendor\Module\Model\PayPal\Express\Checkout"/>
</config>
Step 2: Custom Checkout Model
Create file app/code/Vendor/Module/Model/PayPal/Express/Checkout.php
Add the below-mentioned code:
<?php
namespace Vendor\Module\Model\PayPal\Express;
class Checkout extends \Magento\Paypal\Model\Express\Checkout
{
public function start(
$token,
$returnUrl,
$cancelUrl,
$customerId = null,
$cartId = null
) {
// Customize the amount here
$this->_quote->setBaseGrandTotal($customAmount);
$this->_quote->setGrandTotal($customAmount);
// Call parent method
return parent::start($token, $returnUrl, $cancelUrl, $customerId, $cartId);
}
}
Step 3: Let clear cache and Di Compile
Run the following commands to clear cache and Di Compile:
bin/magento c:f
bin/magento s:d:c
Method 2: Customize the amount in PayPal Express Checkout using an observer to add a custom fee
Step 1: Event Configuration
Create file app/code/Vendor/Extension/etc/events.xml
Add the following content to events.xml:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Event/etc/events.xsd">
<event name="paypal_express_checkout_prepare_line_items">
<observer name="vendor_extension_add_custom_fee" instance="Vendor\Extension\Observer\AddCustomFee"/>
</event>
</config>
Step 2: Observer Class
Create file app/code/Vendor/Extension/Observer/AddCustomFee.php
Step 3: Let clear cache and Di Compile
Run the below code to clear cache and Di Compile:
php bin/magento c:f
php bin/magento s:d:c
<?php
namespace Vendor\Extension\Observer;
use Magento\Framework\Event\Observer;
use Magento\Framework\Event\ObserverInterface;
class AddCustomFee implements ObserverInterface
{
/**
* Execute observer
*
* @param Observer $observer
* @return void
*/
public function execute(Observer $observer)
{
/** @var \Magento\Payment\Model\Cart $cart */
$cart = $observer->getEvent()->getCart();
$customAmount = 100; // Set your custom amount here
$cart->addCustomItem(__('Custom Amount'), 1, -1.00 * $customAmount, 'customfee');
}
}
Important Considerations
- Currency Handling: Ensure the custom amount is correctly handled in the store’s currency.
- Tax Implications: Consider whether the custom amount needs to be taxable.
- Payment Gateway Compliance: Ensure the changes comply with PayPal’s requirements and guidelines.
Summary
By following either of these methods, you can successfully add custom amounts with PayPal Express Checkout in Magento 2. Remember to thoroughly test your implementation to ensure accurate calculations and a seamless checkout experience for your customers.
Related Posts:
How To Install And Configure PayPal For Shopware Store