Magento 2 Get All Order Collection with Filters
During the business management process, Magento 2 stores may have the need to filter all order collections for further analysis. In today’s article I would like to show you how to get all order collection with filters by customer, date, status, and payment method.
Read more about Get Product Collection Filter by Visibility
Method 1: Get All Order Collection with filters
To get all order collections with filters, go to the following path Mageplaza/HelloWorld/Block/Orders.php
and create an Orders.php
file.
<?php
namespace Mageplaza\HelloWorld\Block;
class Products extends \Magento\Framework\View\Element\Template
{
protected $_orderCollectionFactory;
public function __construct(
Magento\Framework\App\Action\Context $context,
\Magento\Sales\Model\ResourceModel\Order\CollectionFactory $orderCollectionFactory
) {
$this->_orderCollectionFactory = $orderCollectionFactory;
parent::__construct($context);
}
public function getOrderCollection()
{
$collection = $this->_orderCollectionFactory->create()
->addAttributeToSelect('*')
->addFieldToFilter($field, $condition); //Add condition if you wish
return $collection;
}
public function getOrderCollectionByCustomerId($customerId)
{
$collection = $this->_orderCollectionFactory()->create($customerId)
->addFieldToSelect('*')
->addFieldToFilter('status',
['in' => $this->_orderConfig->getVisibleOnFrontStatuses()]
)
->setOrder(
'created_at',
'desc'
);
return $collection;
}
}
Method 2: Get All Order Collection Filter by Customer
While developing Magento 2 extensions at the frontend like My Account, you might need to display existing customer orders in a grid in the backend. Down here are how we can get all order filter by customer:
public function getOrderCollectionByCustomerId($customerId)
{
$collection = $this->_orderCollectionFactory()->create($customerId)
->addFieldToSelect('*')
->addFieldToFilter('status',
['in' => $this->_orderConfig->getVisibleOnFrontStatuses()]
)
->setOrder(
'created_at',
'desc'
);
return $collection;
}
Method 3: Get Order Collection Filter by Date
Beside getting an order collection filter by customer, you can also get them by date. And here is how we do it:
public function getOrderCollectionByDate($from, $to)
{
$collection = $this->_orderCollectionFactory()->create($customerId)
->addFieldToSelect('*')
->addFieldToFilter('status',
['in' => $this->_orderConfig->getVisibleOnFrontStatuses()]
)
->setOrder(
'created_at',
'desc'
);
return $collection;
}
Method 4: Get Order Collection Filter by Status
The fourth method to get order collect filters is by status.
public function getOrderCollectionByStatus($statuses = [])
{
$collection = $this->_orderCollectionFactory()->create($customerId)
->addFieldToSelect('*')
->addFieldToFilter('status',
['in' => $statuses]
)
;
return $collection;
}
Method 5: Get Order Collection Filter by Payment Method
Besides the above solutions to help you get order collect filter, there is another way. The following guide will show you how you can get the order collection filter by payment method.
public function getOrderCollectionPaymentMethod($paymentMethod)
{
$collection = $this->_orderCollectionFactory()->create($customerId)
->addFieldToSelect('*')
->addFieldToFilter('created_at',
['gteq' => $from]
)
->addFieldToFilter('created_at',
['lteq' => $to]
);
/* join with payment table */
$collection->getSelect()
->join(
["sop" => "sales_order_payment"],
'main_table.entity_id = sop.parent_id',
array('method')
)
->where('sop.method = ?',$paymentMethod); //E.g: ccsave
$collection->setOrder(
'created_at',
'desc'
);
return $collection;
}
Related Post: How to Add a Custom Column to Order Grid in Magento 2?
Conclusion
Here are 5 simple methods to get all collection of orders which are filtered by different attributes such as customer, date, status, and payment method. I hope after reading this post, you will be able to apply the appropriate method in a specific situation in reality. If you have more ideas on what we should guide you in our next posts, or simply want to share concerns, please drop your comments down below and we will get back to you.
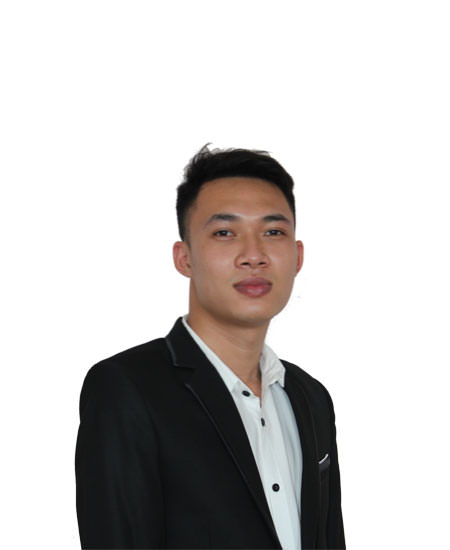
- How to create a simple Hello World module for Magento 2
- Magento 2 Block Template Ultimate Guides
- How to Create Module in Magento 2
- How to Create Controller in Magento 2
- How to create CRUD Models in Magento 2
- How to Create Magento 2 Block, Layout and Templates
- Configuration - System.xml
- How To Create Admin Menu In Magento 2
- Admin ACL
- Admin Grid
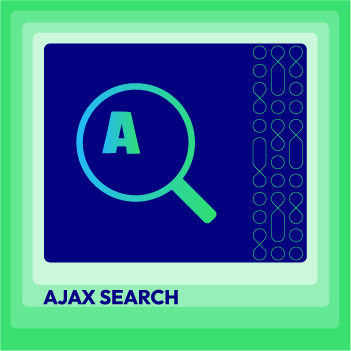
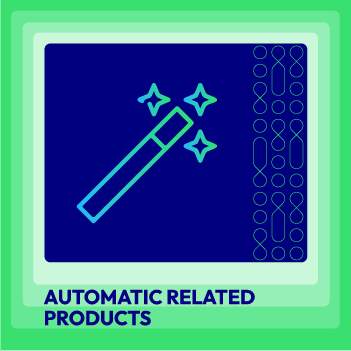
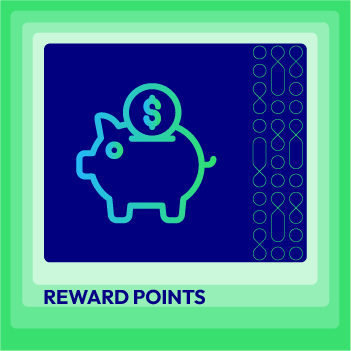
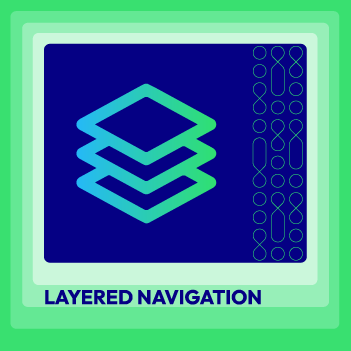
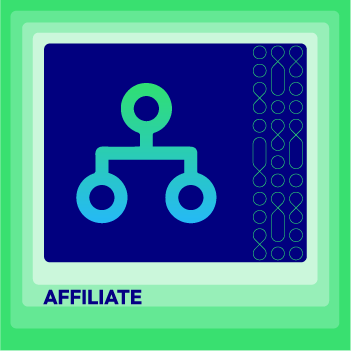
People also searched for
- magento 2 get order collection
- get order collection in magento 2
- magento 2 get all orders
- magento 2 order collection filter by date
- magento 2 order collection filter by status
- magento 2 get order collection filter by date
- magento 2 collection filter
- magento 2 order collection
- order collection magento 2
- magento 2 get all orders programmatically
- magento 2 get order collection by order id
- magento 2 get sales order item collection
- get order magento 2
- magento 2 sort collection
- filter collection magento 2
- magento 2 custom module collection filter
- magento 2 filter collection
- magento 2 get order created date
- magento 2 product collection sort by position
- 2.3.x, 2.4.x