Magento 2 Add Product Attribute Programmatically
Vinh Jacker | 12-18-2024
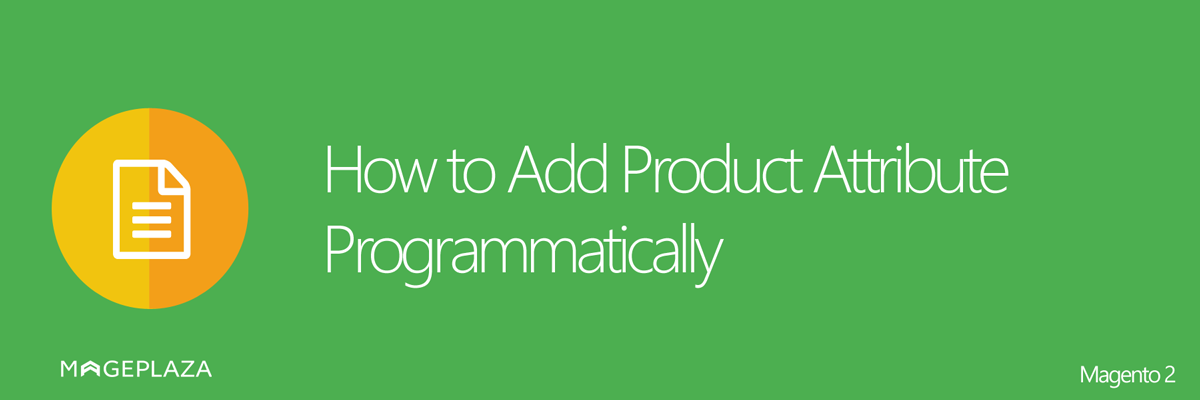
Adding product attributes programmatically in Magento 2 offers flexibility and customization for store owners and developers. This method lets you create new attributes suited to different product types or business needs directly through code, avoiding manual setup in the Magento Admin Panel. Mastering this approach helps streamline store setup, improve product catalog management, and efficiently meet specific business needs. This guide will detail the steps and considerations for adding product attributes programmatically in Magento 2.
Before we start with this, please read the article Magento 2 Install/Upgrade Script to learn how to create a module setup class.
What is A Product Attribute in Magento 2?
A product attribute in Magento 2 is a property or characteristic assigned to a product. These attributes include elements like size, color, weight, and other descriptive details. Attributes help customers filter and search for products based on specific features, making it easier for them to find what they are looking for.
Attributes are a key part of your product catalog, offering not just improved categorization but also enabling you to create detailed, organized product descriptions. Magento 2 allows both system (default) attributes and custom attributes, which you can create based on your unique store requirements.
Why We Need to Add Product Attribute Programmatically Magento 2?
Programmatic attribute creation in Magento 2 offers several advantages:
- Efficiency in Bulk Actions: Manually adding attributes through the admin panel can be time-consuming, especially with many attributes. Automating this process saves significant time and effort.
- Integration with External Data Sources: If your product data originates from external systems like CRM or ERP, automating attribute creation based on this data streamlines integration.
- Conditional Attribute Creation: Customize attribute creation based on specific criteria. For example, attributes can be created only for products in designated categories.
- Version Control: Incorporate attribute creation scripts into version control systems, simplifying tracking and management of code changes.
Steps to Add Product Attribute Programmatically in Magento 2
Now, we will use the Mageplaza HelloWorld module to learn how to add a product attribute.
Step 1: UpgradeAttributeData.php
We will start with the InstallData class which located in app/code/Mageplaza/HelloWorld/Setup/InstallData.php
. The content for this file:
<?php
namespace Mageplaza\HelloWorld\Setup\Patch\Data;
use Magento\Catalog\Model\Category;
use Magento\Eav\Model\Entity\Attribute\ScopedAttributeInterface;
use Magento\Framework\Setup\ModuleDataSetupInterface;
use Magento\Framework\Setup\Patch\DataPatchInterface;
use Magento\Framework\Setup\Patch\PatchRevertableInterface;
use Magento\Eav\Setup\EavSetupFactory;
class UpgradeAttributeData implements
DataPatchInterface,
PatchRevertableInterface
{
private $moduleDataSetup;
private $eavSetupFactory;
public function __construct(
ModuleDataSetupInterface $moduleDataSetup,
EavSetupFactory $eavSetupFactory
) {
$this->moduleDataSetup = $moduleDataSetup;
$this->eavSetupFactory = $eavSetupFactory;
}
}
Step 2: Define the apply(), revert(), getAliases(), getDependencies() method
public function apply()
{
}
public function revert()
{
}
public function getAliases()
{
return [];
}
public static function getDependencies()
{
return [];
}
Step 3: Create custom attribute in apply() method
Here are all lines code of UpgradeAttributeData.php to create product attribute programmatically.
<?php
namespace Mageplaza\HelloWorld\Setup\Patch\Data;
use Magento\Catalog\Model\Category;
use Magento\Eav\Model\Entity\Attribute\ScopedAttributeInterface;
use Magento\Framework\Setup\ModuleDataSetupInterface;
use Magento\Framework\Setup\Patch\DataPatchInterface;
use Magento\Framework\Setup\Patch\PatchRevertableInterface;
use Magento\Eav\Setup\EavSetupFactory;
class UpgradeAttributeData implements
DataPatchInterface,
PatchRevertableInterface
{
private $moduleDataSetup;
private $eavSetupFactory;
public function __construct(
ModuleDataSetupInterface $moduleDataSetup,
EavSetupFactory $eavSetupFactory
) {
$this->moduleDataSetup = $moduleDataSetup;
$this->eavSetupFactory = $eavSetupFactory;
}
public function apply()
{
$setup = $this->moduleDataSetup;
$eavSetup = $this->eavSetupFactory->create(['setup' => $setup]);
$eavSetup->addAttribute(Category::ENTITY, 'sample_attribute', [
'type' => 'text',
'label' => 'Sample Atrribute',
'input' => 'text',
'source' => '',
'backend' => '',
'required' => false,
'sort_order' => 100,
'global' => ScopedAttributeInterface::SCOPE_STORE,
'group' => '',
]);
$setup->endSetup();
}
public function revert()
{
}
public function getAliases()
{
return [];
}
public static function getDependencies()
{
return [];
}
}
As you can see, all the addAttribute method requires is:
- The type ID of the entity which we want to add attribute
- The name of the attribute
- An array of key-value pairs to define the attribute such as group, input type, source, label…
All done, please run the upgrade script php bin/magento setup:upgrade
to install the module and the product attribute sample_attribute
will be created. After run upgrade
complete, please run php bin/magento setup:static-content:deploy
and go to product from admin to check the result. it will show like this:
If you want to remove product attribute, you can use method removeAttribute instead of addAttribute. It will be like this:
public function install(ModuleDataSetupInterface $setup, ModuleContextInterface $context)
{
$eavSetup = $this->eavSetupFactory->create(['setup' => $setup]);
$eavSetup->removeAttribute(
\Magento\Catalog\Model\Product::ENTITY,
'sample_attribute');
}
Conclusion
Product attributes are a powerful tool in Magento 2, enabling you to customize your catalog and improve the customer experience. By following the steps in this guide, you can easily add new product attributes and ensure that they are effectively organized within your store. Regularly managing and optimizing your attributes will help your store stay organized, provide customers with a better shopping experience, and even boost your SEO.
Related Topics