The Ultimate Guide to Use Curl in Magento 2
Vinh Jacker | 09-17-2024
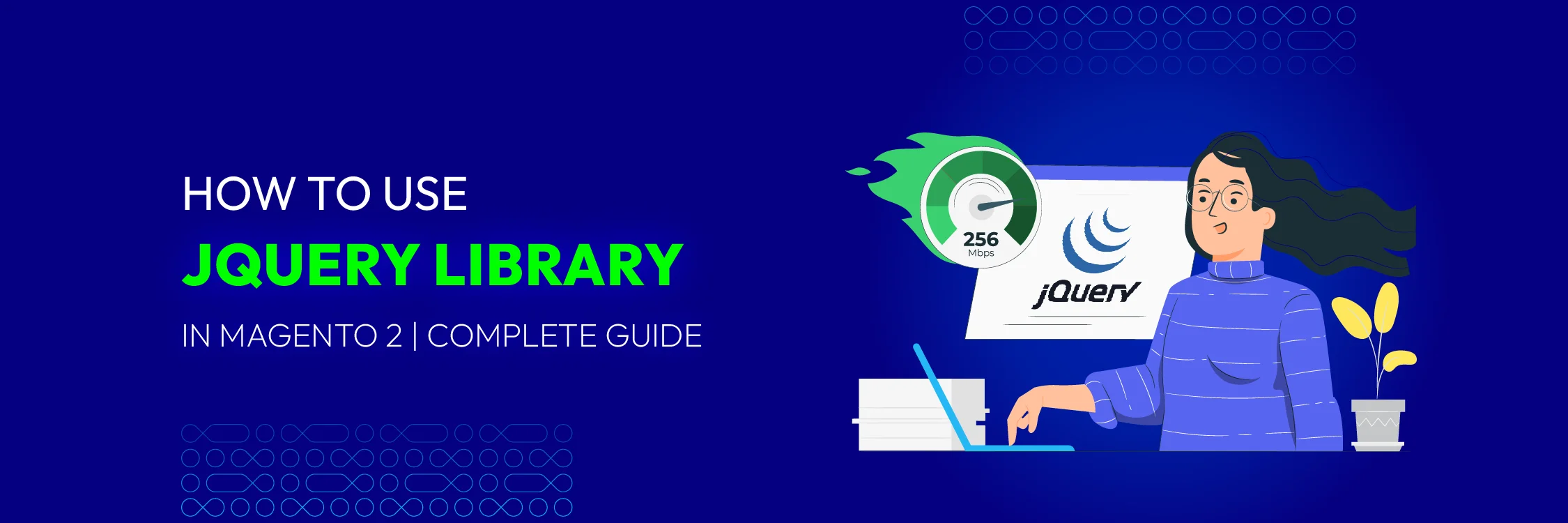
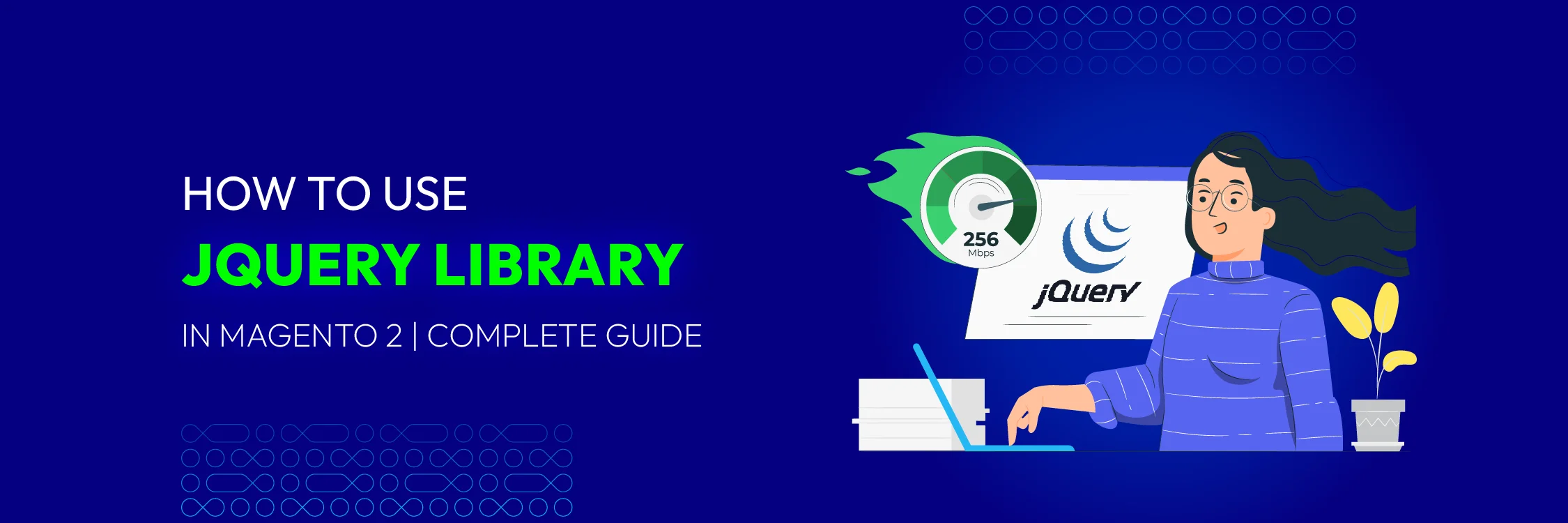
The Most Popular Extension Builder for Magento 2
With a big catalog of 224+ extensions for your online store
Magento 2 is a useful tool for creating and managing online stores. To enhance its capabilities, it often needs to connect with external services, APIs, or other servers. This is when cURL becomes necessary. cURL allows Magento 2 developers to perform HTTP requests programmatically, enabling them to transfer data from other sources or send data to them. In this article, we will explore how to use cURL in Magento 2.
Create an Instance in Constructor
First of all, it is necessary to create an instance of \Magento\Framework\HTTP\Client\Curl
within Constructor as illustrated below:
/**
* @var \Magento\Framework\HTTP\Client\Curl
*/
protected $_curl;
/**
* Data constructor.
*
* @param \Magento\Framework\App\Helper\Context $context
* @param \Magento\Framework\HTTP\Client\Curl $curl
*/
public function __construct(
\Magento\Framework\App\Helper\Context $context,
\Magento\Framework\HTTP\Client\Curl $curl
) {
$this->_curl = $curl;
parent::__construct($context);
}
Send GET Request
For GET requests, use the below-mentioned code:
//get method
$this->_curl->get($url);
//response will contain the output of curl request
$response = $this->_curl->getBody();
Send POST Request
Use the following code snippet to make a POST request:
//post method
$this->_curl->post($url, $params);
//response will contain the output of curl request
$response = $this->_curl->getBody();
In the above code, $url
holds the endpoint URL, $params
is an array for extra URL parameters, and $response
contains the curl request response.
Besides, you can add headers, basic authorization, additional cURL options, and cookies in your cURL request. You need to ensure these methods are set before using GET or POST method. Detailed instructions on how to implement these additions are provided below.
Set cURL Headers
You can set cURL headers using either addHeader or setHeaders methods.
Use addHeader method to set cURL header
The addheader method accepts two parameters. They are the cURL header name and the cURL header value.
$this->_curl->addHeader("Content-Type", "application/json");
$this->_curl->addHeader("Content-Length", 200);
Use setHeaders method to set cURL header
The setHeaders method is designed to accept one parameter, specifically in the form of an array.
$headers = ["Content-Type" => "application/json", "Content-Length" => "200"];
$this->_curl->setHeaders($headers);
Set Basic Authorization
You can set the basic authorization by using the setCredentials
method.
$userName = "UserName";
$password = "Password";
$this->_curl->setCredentials($userName, $password);
This code is equivalent to setting the CURLOPT_HTTPHEADER
value to
“Authorization : “. “Basic “.base64_encode($userName.”:”.$password)
Set Additional Curl Options
There are two methods to set the cURL options, including setOption and setOptions.
Use setOption method to set cURL option
The setOption method permits the assignment of individual cURL options by accepting two parameters. They are the cURL option name and the option value.
$this->_curl->setOption(CURLOPT_RETURNTRANSFER, true);
$this->_curl->setOption(CURLOPT_PORT, 8080);
Use setOptions method to set cURL option
The setOptions method accepts one parameter, specifically in the form of an array.
$options = [CURLOPT_RETURNTRANSFER => true, CURLOPT_PORT => 8080];
$this->_curl->setOptions($options);
Set Cookies in Curl
There are two methods to set the cURL cookies in cURL, including addCookie and setCookies.
Use addCookie method to Set cURL cookies
The addCookie method accepts two parameters. They are the cookie name and the cookie value.
$this->_curl->addCookie("cookie-name-1", "123");
$this->_curl->addCookie("cookie-name-2", "test-cookie-value");
Use setCookies method to set cURL cookies
The setCookies method accepts one parameter, specifically in the form of an array.
$cookies = ["cookie-name-1" => "100", "cookie-name-2" => "name"];
$this->_curl->setCookies($cookies);
Conclusion
Understanding how to use cURL in Magento 2 opens up numerous possibilities for integrating external services, and enhancing the functionality of your e-commerce store. By following the steps in this guide, you can efficiently handle HTTP requests and ensure seamless data exchange between your Magento 2 store and external APIs.